Optimizing Database Performance: A Practical Guide for Modern Applications
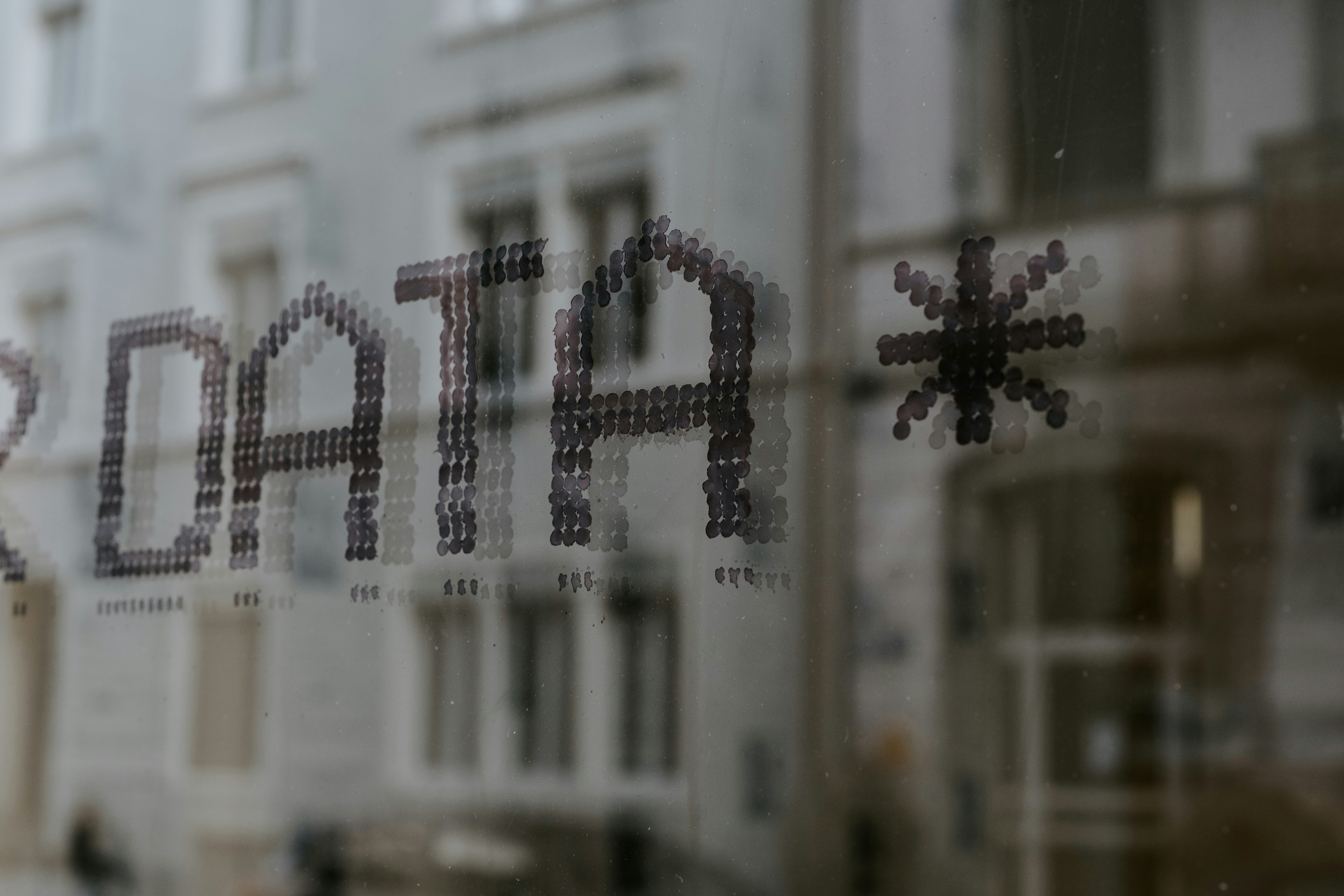
Optimizing Database Performance: A Practical Guide for Modern Applications
In the world of application development, database performance often makes the difference between a responsive, scalable system and one that frustrates users with delays and outages. As data volumes grow and user expectations for speed increase, optimizing database performance has become more critical than ever.
Having designed and optimized databases across multiple organizations and industries, I’ve seen firsthand how the right approach to database architecture can dramatically improve application performance, user satisfaction, and system scalability. This article shares practical strategies for database optimization based on real-world experience.
Why Database Performance Matters
Before diving into optimization techniques, it’s worth understanding why database performance deserves special attention:
- User Experience Impact: Database delays directly translate to application lag that users experience.
- Scalability Limitations: Inefficient databases often become bottlenecks that prevent application scaling.
- Resource Utilization: Optimized databases require less hardware, reducing infrastructure costs.
- Downstream Effects: Poor database performance can cascade through systems, causing unpredictable issues.
Modern applications may have beautiful interfaces and sophisticated features, but their success ultimately depends on the speed and reliability of data access. Let’s explore how to ensure your database delivers the performance your application needs.
Foundation: Data Modeling for Performance
Database optimization starts long before the first query runs—it begins with data modeling:
1. Balancing Normalization and Performance
While database normalization eliminates redundancy and improves data integrity, it can also increase query complexity:
- Thoughtful Denormalization: Strategically relax normalization rules for performance-critical data.
- Derived Data: Store calculated values when real-time calculation would be expensive.
- Aggregation Tables: Maintain summary tables for frequently accessed metrics.
-- Example of a pre-calculated aggregation table
CREATE TABLE monthly_sales_summary (
month DATE,
product_id INT,
total_quantity INT,
total_revenue DECIMAL(10,2),
average_unit_price DECIMAL(10,2),
PRIMARY KEY (month, product_id)
);
The key is striking the right balance—normalize enough to maintain data integrity while denormalizing where performance demands it.
2. Appropriate Data Types and Sizes
Choosing the right data types seems simple but has significant performance implications:
- Size Appropriately: Use the smallest data type that accommodates your needs (e.g., TINYINT vs INT when appropriate).
- Fixed vs Variable Length: Consider performance implications of VARCHAR vs CHAR.
- Specialized Types: Use types designed for specific data (e.g., DATE instead of strings for dates).
For a client with over 100 million records, simply changing a legacy VARCHAR(255) field to a more appropriate VARCHAR(50) reduced storage requirements by 40GB and noticeably improved query performance.
3. Logical Data Grouping
How you group related data affects access patterns:
- Access Pattern Analysis: Understand which data is typically accessed together.
- Table Partitioning: Group related rows physically for more efficient access.
- Vertical Splitting: Separate frequently and rarely accessed columns.
In one optimization project, we moved rarely accessed large text fields to a separate table, reducing the main table size by 60% and improving common query performance by 45%.
Query Optimization: Where Performance Meets Code
Even with excellent data modeling, poorly written queries can devastate performance:
1. Identify Performance-Critical Queries
Not all queries deserve equal optimization effort:
- Query Frequency: Focus on queries executed most often.
- Execution Time: Prioritize long-running queries.
- Business Impact: Consider the operational importance of affected functionality.
Tools like slow query logs and performance monitoring help identify where optimization efforts will yield the greatest returns.
2. Use Execution Plan Analysis
Database query planners provide valuable insights:
- EXPLAIN Command: Use your database’s explain functionality to understand query execution.
- Identify Table Scan Issues: Look for full table scans on large tables.
- Join Order Problems: Check if the optimizer is choosing efficient join sequences.
- Index Usage: Confirm queries are using available indexes appropriately.
-- Example MySQL EXPLAIN analysis
EXPLAIN SELECT customer_id, COUNT(*) as order_count
FROM orders
WHERE order_date > '2024-01-01'
GROUP BY customer_id
HAVING COUNT(*) > 5;
This analysis often reveals simple changes that can yield dramatic performance improvements.
3. Common Query Optimization Techniques
Several patterns appear frequently in query optimization:
- Limit Result Sets: Only retrieve the data you actually need.
- *Avoid SELECT : Specify required columns explicitly.
- Join Optimization: Ensure efficient join conditions and proper table ordering.
- Subquery Refactoring: Convert problematic subqueries to joins when possible.
- LIMIT with Pagination: Use efficient pagination for large result sets.
-- Before optimization
SELECT * FROM orders o
JOIN customers c ON o.customer_id = c.id
WHERE YEAR(o.order_date) = 2024;
-- After optimization
SELECT o.id, o.order_date, o.total_amount, c.name, c.email
FROM orders o
JOIN customers c ON o.customer_id = c.id
WHERE o.order_date >= '2024-01-01' AND o.order_date < '2025-01-01';
Notice how the optimized query specifies exact columns, avoids function calls on indexed fields, and uses range conditions that can leverage indexes.
Indexing Strategy: The Performance Multiplier
Proper indexing can improve query performance by orders of magnitude, but requires careful planning:
1. Fundamental Indexing Principles
Effective indexing follows several key principles:
- Index for Queries, Not Tables: Base indexing decisions on actual query patterns.
- High Selectivity: Index columns that filter out significant portions of data.
- Query Frequency: Prioritize indexes for commonly executed queries.
- Write Impact: Consider the performance cost on write operations.
Before adding an index, always ask: “Will this index be used frequently enough to justify its maintenance cost?“
2. Composite Index Design
Multi-column indexes require special consideration:
- Column Order Matters: Place higher selectivity columns first in composite indexes.
- Consider Query Patterns: Design composite indexes that support multiple queries.
- Covering Indexes: Include all columns referenced in queries to enable index-only scans.
-- Composite index supporting multiple query patterns
CREATE INDEX idx_orders_customer_date ON orders (customer_id, order_date, status);
-- This index helps all these queries:
-- SELECT * FROM orders WHERE customer_id = 123;
-- SELECT * FROM orders WHERE customer_id = 123 AND order_date > '2024-01-01';
-- SELECT * FROM orders WHERE customer_id = 123 AND order_date > '2024-01-01' AND status = 'shipped';
The example above demonstrates a single index that can support multiple query variations, maximizing the index’s utility.
3. Index Maintenance and Monitoring
Indexes require ongoing attention:
- Usage Analysis: Regularly review which indexes are being used.
- Duplicate/Redundant Index Identification: Remove unnecessary indexes that increase write overhead.
- Index Fragmentation: Rebuild indexes that have become fragmented over time.
- Missing Index Detection: Use database advisor tools to identify beneficial indexes.
For one e-commerce client, removing seven redundant indexes reduced their daily batch processing time by 35% while maintaining fast query response times.
Scaling Strategies for Growing Databases
As data volumes grow, additional strategies become necessary:
1. Partitioning for Large Tables
Table partitioning can dramatically improve performance for large datasets:
- Horizontal Partitioning: Split tables by row based on value ranges (e.g., date ranges).
- Vertical Partitioning: Split tables by column based on access patterns.
- Partition Pruning: Ensure queries can efficiently target specific partitions.
-- Example of date-based partitioning in MySQL
CREATE TABLE orders (
id INT,
customer_id INT,
order_date DATE,
total_amount DECIMAL(10,2),
-- other columns...
PRIMARY KEY (id, order_date)
)
PARTITION BY RANGE (YEAR(order_date)) (
PARTITION p2022 VALUES LESS THAN (2023),
PARTITION p2023 VALUES LESS THAN (2024),
PARTITION p2024 VALUES LESS THAN (2025),
PARTITION future VALUES LESS THAN MAXVALUE
);
This approach allows the database to quickly eliminate irrelevant partitions during query execution, dramatically reducing I/O.
2. Caching Strategies
Caching reduces database load for frequently accessed data:
- Application-level Caching: Store commonly accessed data in application memory.
- Database Query Cache: Leverage database-specific query caching features.
- Distributed Caching: Implement solutions like Redis or Memcached for multi-server environments.
- Cache Invalidation Strategy: Ensure cached data remains current without excessive refreshing.
A thoughtful caching strategy can reduce database load by 70-80% for read-heavy applications, as we observed when implementing Redis caching for a high-traffic e-commerce site.
3. Read/Write Splitting
For high-volume applications, separating read and write operations can improve scalability:
- Master-Slave Replication: Direct writes to the master and reads to replicas.
- Query Routing: Intelligently route queries based on their nature.
- Consistency Considerations: Manage potential data staleness in read replicas.
This approach allowed one of my clients to scale their application to handle 5x their previous traffic without changing their core database architecture.
Performance Monitoring and Tuning
Optimization is an ongoing process requiring visibility and measurement:
1. Establish Performance Baselines
You can’t improve what you don’t measure:
- Key Metrics: Track query execution times, resource utilization, and throughput.
- Regular Benchmarking: Periodically test performance against standard workloads.
- Trend Analysis: Monitor performance changes over time.
These baselines provide context for identifying performance regressions and measuring improvements.
2. Implement Monitoring Systems
Proactive monitoring helps catch issues before they impact users:
- Query Performance Monitoring: Track execution times and resource usage.
- Resource Utilization: Monitor CPU, memory, disk I/O, and network metrics.
- Wait Event Analysis: Identify bottlenecks through database wait events.
- Alerting: Set up notifications for abnormal performance conditions.
Tools like Prometheus, Grafana, and database-specific monitoring solutions provide visibility into database health and performance.
3. Regular Maintenance Practices
Databases require ongoing maintenance for optimal performance:
- Statistics Updates: Ensure query optimizers have current data distribution information.
- Index Maintenance: Rebuild fragmented indexes periodically.
- Configuration Tuning: Adjust database parameters based on observed behavior.
- Query Plan Analysis: Review execution plans for critical queries after significant data changes.
These maintenance practices help prevent gradual performance degradation as data and access patterns evolve.
Real-World Optimization Case Study
To illustrate these principles in action, here’s a brief case study from my experience:
An e-commerce platform was experiencing slow performance during peak shopping periods. Customer order processing had grown from 2 seconds to over 15 seconds, causing abandoned carts and lost revenue.
Key Issues Identified:
- Complex joins across multiple tables in the order processing query
- Missing indexes on frequently filtered columns
- Inefficient date handling using functions that prevented index usage
- Redundant data fetching in application code
Optimization Approach:
- Restructured the order query to eliminate unnecessary joins
- Added composite indexes based on actual query patterns
- Refactored date filtering to enable index usage
- Implemented application-level caching for product catalog data
- Created a denormalized order summary table for reporting queries
Results:
- Order processing time reduced from 15+ seconds to under 1 second
- Peak database server load decreased by 65%
- Able to handle 3x previous traffic volume without hardware upgrades
- Reporting query performance improved by 80%
This example demonstrates how combining multiple optimization techniques—query refactoring, proper indexing, caching, and strategic denormalization—can transform database performance.
Conclusion: Performance by Design
Database optimization is not a one-time task but an ongoing process that should be integrated into your development and operations practices. The most successful organizations build performance considerations into their database design from the beginning and continuously monitor and refine as applications evolve.
By focusing on thoughtful data modeling, query optimization, strategic indexing, and appropriate scaling strategies, you can create database systems that deliver the performance your applications need while adapting to growing data volumes and user demands.
Remember that database optimization isn’t just about technical excellence—it’s about delivering better user experiences, enabling business growth, and creating systems that scale efficiently as your organization evolves.
What database performance challenges is your organization facing? I’d love to hear about your experiences in the comments below.
Need help optimizing your database performance? Contact me to discuss how I can help your organization create efficient, scalable database architectures that support your business objectives.
About the Author: Anthony Trivisano is a database architecture specialist with extensive experience designing and optimizing database systems across multiple industries. He has successfully transformed database performance for organizations ranging from e-commerce platforms to financial institutions, creating scalable solutions that balance data integrity with query performance.