Database Optimization Strategies for Modern Web Applications
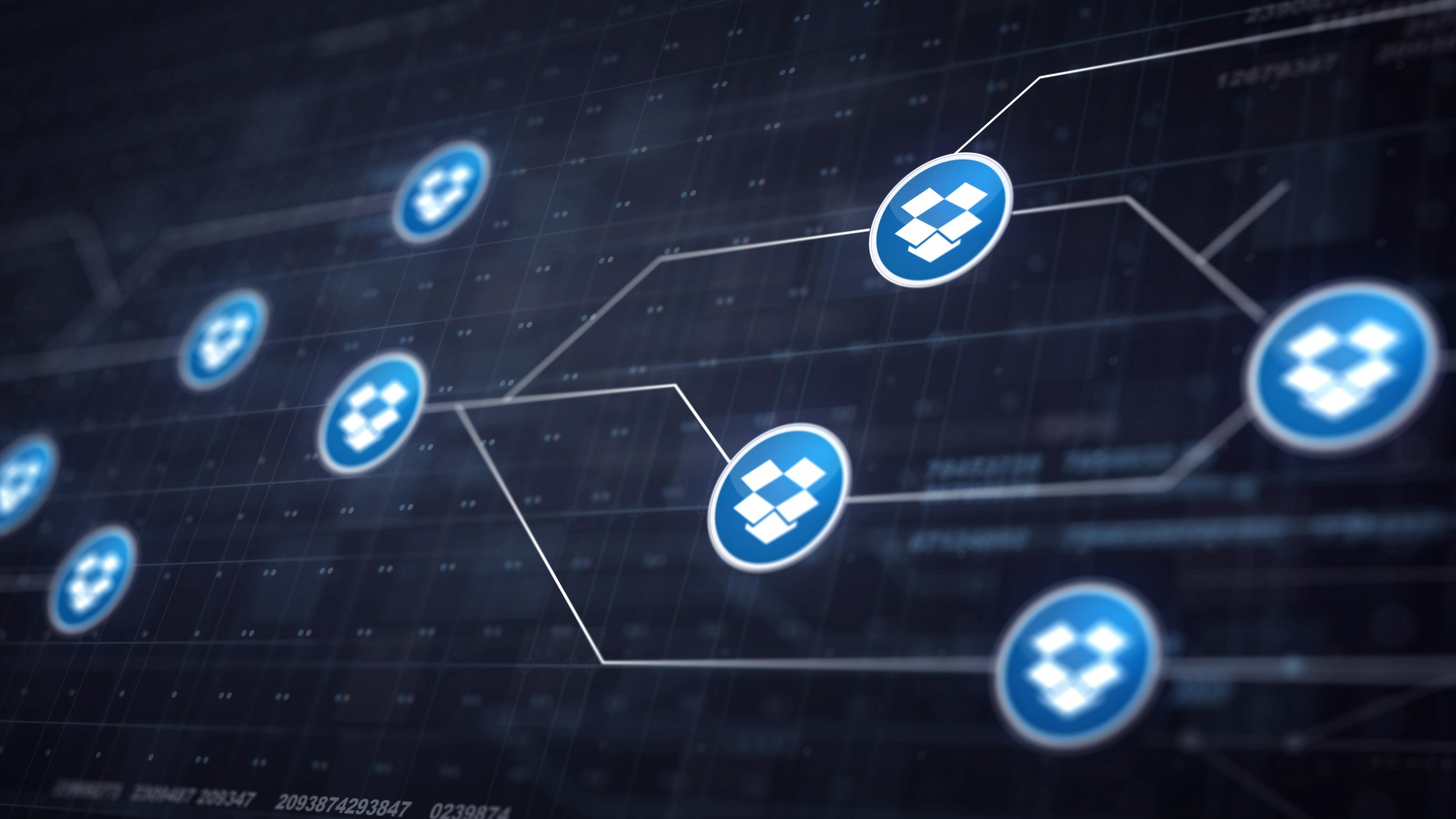
Database Optimization Strategies for Modern Web Applications
Database performance remains one of the most critical factors in web application success. No matter how efficient your frontend code or how elegant your architecture, a poorly optimized database can bring your entire application to a crawl, frustrating users and limiting scalability.
Over my years of designing and optimizing database systems for organizations ranging from startups to large enterprises, I’ve found that database performance problems often share similar patterns—and respond to similar solutions. In this article, I’ll explore proven strategies for database optimization that can dramatically improve web application performance, scalability, and reliability.
Understanding Database Performance Bottlenecks
Before diving into specific optimization techniques, it’s crucial to understand the common causes of database performance issues in web applications:
Query Inefficiency
Poorly structured queries are often the primary culprit behind database performance problems:
- Excessive data retrieval: Fetching more data than needed
- Suboptimal join operations: Joining tables inefficiently or unnecessarily
- Missing or improper indexes: Forcing the database to scan entire tables
- N+1 query problems: Executing multiple separate queries where a single query would suffice
- Inappropriate aggregation methods: Performing calculations that could be pre-computed or simplified
Architectural Limitations
Sometimes the database design itself imposes fundamental limitations:
- Normalization vs. denormalization imbalance: Over-normalized schemas requiring excessive joins, or under-normalized schemas causing data inconsistency
- Poor data modeling: Tables and relationships that don’t align with actual query patterns
- Monolithic database approach: Using a single database for all operations regardless of workload characteristics
- Inadequate partitioning: Not dividing large tables to improve manageability and query performance
- Ineffective caching strategies: Missing opportunities to reduce database load
Resource Constraints
Physical resource limitations can become bottlenecks as applications scale:
- Insufficient memory: Inadequate RAM for query execution and caching
- I/O bottlenecks: Disk performance limitations affecting read/write operations
- CPU constraints: Processor limitations impacting query execution
- Network latency: Delays in data transmission between database and application servers
- Connection management issues: Inefficient handling of database connections
Core Optimization Strategies
Let’s explore key strategies to address these bottlenecks, starting with the most foundational approaches.
1. Query Optimization
Query optimization often provides the most immediate performance improvements:
Selective Data Retrieval
Always fetch only the data you actually need:
-- Instead of this:
SELECT * FROM users JOIN orders ON users.id = orders.user_id;
-- Do this:
SELECT users.id, users.name, orders.order_date, orders.total
FROM users JOIN orders ON users.id = orders.user_id
WHERE orders.created_at > '2024-01-01';
Benefits:
- Reduces I/O operations and memory usage
- Decreases network traffic between database and application
- Improves query execution time
- Reduces load on the application server
Index Optimization
Proper indexing dramatically improves query performance:
-- Create an index on frequently queried columns
CREATE INDEX idx_orders_user_id_created_at
ON orders(user_id, created_at);
-- Create a covering index for common queries
CREATE INDEX idx_users_email_name_status
ON users(email, name, status);
When designing indexes:
- Create indexes on columns used in WHERE, JOIN, and ORDER BY clauses
- Consider covering indexes that include all columns needed by frequent queries
- Be cautious with over-indexing, as it impacts write performance
- Regularly analyze index usage and remove unused indexes
- Consider partial indexes for large tables where only a subset of rows is frequently queried
Efficient Joins
Optimize how tables are joined:
-- Ensure proper join types
SELECT p.title, c.name
FROM posts p
LEFT JOIN comments c ON p.id = c.post_id
WHERE p.published = true;
-- Use subqueries or CTEs when appropriate
WITH active_users AS (
SELECT id, name FROM users WHERE status = 'active'
)
SELECT au.name, COUNT(o.id) as order_count
FROM active_users au
JOIN orders o ON au.id = o.user_id
GROUP BY au.id, au.name;
Best practices:
- Join on indexed columns
- Choose appropriate join types (INNER, LEFT, RIGHT) based on data requirements
- Consider denormalizing when join performance becomes prohibitive
- Use EXISTS instead of IN for better performance with large datasets
- Consider JOIN elimination by adding necessary columns to indexes
Query Rewriting
Restructure queries for improved performance:
- Replace subqueries with joins when possible
- Use window functions instead of multiple self-joins
- Leverage CTEs (Common Table Expressions) for complex queries
- Transform OR conditions to UNION ALL operations when advantageous
- Use EXPLAIN to analyze query execution plans and identify optimization opportunities
2. Schema Optimization
The database schema provides the foundation for performance:
Strategic Denormalization
While normalization is a best practice for data integrity, strategic denormalization can significantly improve read performance:
-- Normalized approach (requires a join)
SELECT users.name, addresses.city, addresses.country
FROM users
JOIN addresses ON users.id = addresses.user_id;
-- Denormalized approach (no join needed)
SELECT name, city, country FROM users;
Consider denormalization when:
- Read performance is more critical than write performance
- Join operations become prohibitively expensive
- Redundant data can be kept consistent through application logic or triggers
- The data rarely changes
Appropriate Data Types
Choose the most efficient data types for your data:
-- Instead of:
CREATE TABLE products (
id VARCHAR(36), -- UUID as string
name TEXT, -- Unlimited length
price FLOAT, -- Floating point
details JSON -- Arbitrary JSON
);
-- Consider:
CREATE TABLE products (
id BINARY(16), -- More efficient UUID storage
name VARCHAR(100), -- Limited length
price DECIMAL(10,2), -- Fixed precision
details JSONB -- Binary JSON with indexing support
);
Guidelines:
- Use the smallest data type that accommodates your data needs
- Choose fixed-length types when the length is consistent
- Use specialized types (UUID, JSON, ARRAY) when supported by your database
- Consider storage and computational efficiency in type selection
- Be aware of how different types impact indexing strategies
Table Partitioning
For large tables, partitioning improves both performance and manageability:
-- PostgreSQL example of partitioning by date range
CREATE TABLE orders (
id INT,
user_id INT,
order_date DATE,
amount DECIMAL(10,2)
) PARTITION BY RANGE (order_date);
CREATE TABLE orders_2023 PARTITION OF orders
FOR VALUES FROM ('2023-01-01') TO ('2024-01-01');
CREATE TABLE orders_2024 PARTITION OF orders
FOR VALUES FROM ('2024-01-01') TO ('2025-01-01');
Benefits:
- Queries can skip partitions that aren’t relevant
- Maintenance operations can target specific partitions
- Separate storage strategies can be applied to different partitions
- Archiving old data becomes simpler
3. Indexing Strategies
Effective indexing is perhaps the most crucial aspect of database optimization:
Index Types and Selection
Different types of indexes serve different purposes:
- B-tree indexes: General-purpose indexes ideal for equality and range conditions
- Hash indexes: Optimized for equality comparisons only
- Bitmap indexes: Efficient for low-cardinality columns (few distinct values)
- GIN/GiST indexes: Specialized for full-text search, array operations, and complex data types
- Spatial indexes: Optimized for geographic data
- Partial indexes: Index only a subset of rows based on a condition
- Covering indexes: Include all columns needed by a query to avoid table lookups
When choosing indexes:
- Identify the most frequent and performance-critical queries
- Analyze the WHERE, JOIN, and ORDER BY clauses in these queries
- Consider the cardinality (number of distinct values) of potential index columns
- Balance read performance gains against write performance impacts
- Monitor and revise indexes based on actual usage patterns
Composite Indexes
Composite indexes on multiple columns can significantly improve performance:
-- Create a composite index on commonly filtered columns
CREATE INDEX idx_products_category_brand
ON products(category_id, brand_id);
Important considerations:
- Column order matters – place columns used in equality conditions before those used in range conditions
- Leftmost prefix rule applies (an index on (A,B,C) can be used for queries filtering on A, A+B, or A+B+C, but not B alone)
- Avoid duplicating indexes (an index on (A,B) makes an index on just (A) redundant)
- Consider the selectivity of each column when determining order
Index Maintenance
Indexes require ongoing attention:
- Regularly rebuild indexes to reduce fragmentation
- Remove unused indexes that only slow down write operations
- Update statistics to ensure the query optimizer makes good decisions
- Consider adding or modifying indexes as query patterns evolve
- Be aware of how database operations like bulk loading affect indexes
4. Caching Strategies
Caching reduces database load by serving frequently accessed data from memory:
Multi-Level Caching
Implement caching at multiple levels:
┌─────────────────────────────────────────┐
│ │
│ Client-Side Cache │
│ │
└─────────────────┬───────────────────────┘
│
▼
┌─────────────────────────────────────────┐
│ │
│ Application-Level Cache │
│ │
└─────────────────┬───────────────────────┘
│
▼
┌─────────────────────────────────────────┐
│ │
│ Database Cache │
│ │
└─────────────────────────────────────────┘
- Client-side caching: Browser storage, HTTP caching
- Application-level caching: In-memory caches, distributed cache systems
- Database caching: Query cache, buffer pool, result caching
- Content Delivery Networks: For globally distributed applications
Caching Patterns
Different caching approaches suit different scenarios:
- Cache-aside pattern: Application checks cache first, retrieves from database if not found, then updates cache
- Read-through caching: Cache automatically loads data from database when not found
- Write-through caching: Updates are written to both cache and database
- Write-behind caching: Updates are written to cache and asynchronously persisted to database
- Refresh-ahead caching: Proactively refresh cache entries before expiration
Cache Invalidation Strategies
Determine how to keep cached data fresh:
- Time-based expiration: Cache entries expire after a fixed period
- Event-based invalidation: Cache entries are invalidated when underlying data changes
- Version-based invalidation: Cache keys include a version that changes with updates
- Write-invalidate approach: Write operations invalidate related cache entries
- Soft vs. hard invalidation: Decide whether stale data is acceptable temporarily
Advanced Scaling Patterns
As applications grow, more sophisticated approaches become necessary.
1. Vertical vs. Horizontal Scaling
Understand when to scale up versus scale out:
Vertical Scaling (Scale Up):
- Adding more resources (CPU, RAM, storage) to existing database servers
- Advantages: Simpler architecture, no distribution complexity
- Limitations: Hardware ceiling, single point of failure, cost inefficiency at scale
Horizontal Scaling (Scale Out):
- Adding more database servers to distribute the load
- Advantages: Theoretically unlimited scaling, improved fault tolerance
- Challenges: More complex architecture, data consistency issues, application changes
Most successful large-scale applications use a combination of both approaches.
2. Read/Write Splitting
Distribute read and write operations across specialized instances:
┌───────────────────┐ ┌───────────────────┐
│ │ │ │
│ Write Workload │ │ Read Workload │
│ │ │ │
└────────┬──────────┘ └─────────┬─────────┘
│ │
▼ ▼
┌───────────────────┐ ┌───────────────────┐
│ │ │ │
│ Primary Database ├─────►│ Replica Database │
│ │ │ │
└───────────────────┘ └───────────────────┘
- Direct all write operations to a primary database
- Route read operations to read replicas
- Configure replication to balance consistency vs. latency
- Implement connection pooling to manage connections efficiently
- Use load balancers to distribute read queries across replicas
3. Sharding
Partition data horizontally across multiple database instances:
┌───────────────────────────────────────────────────┐
│ │
│ Application │
│ │
└─────────────┬───────────────────────┬─────────────┘
│ │
▼ ▼
┌─────────────────────┐ ┌─────────────────────┐
│ │ │ │
│ Shard 1 (user_id │ │ Shard 2 (user_id │
│ 1-1,000,000) │ │ 1,000,001-2M) │
│ │ │ │
└─────────────────────┘ └─────────────────────┘
Sharding strategies:
- Range-based sharding: Partition data based on value ranges
- Hash-based sharding: Distribute data using a hash function
- Geographic sharding: Partition data by geographic regions
- Directory-based sharding: Use a lookup service to track data location
- Entity-group sharding: Keep related entities on the same shard
Challenges to address:
- Cross-shard transactions and joins
- Rebalancing data across shards
- Schema changes across all shards
- Maintaining a global unique ID strategy
- Handling varying shard sizes (hot spots)
4. Polyglot Persistence
Use different database types for different workloads:
┌───────────────────────────────────────────────────┐
│ │
│ Application │
│ │
└────┬────────────────┬───────────────────┬─────────┘
│ │ │
▼ ▼ ▼
┌──────────┐ ┌──────────────┐ ┌────────────┐
│ │ │ │ │ │
│ Relational│ │ Document │ │ Graph │
│ Database │ │ Database │ │ Database │
│ │ │ │ │ │
└──────────┘ └──────────────┘ └────────────┘
Common combinations:
- Relational databases for transactional data
- Document databases for unstructured content
- Graph databases for highly connected data
- Time-series databases for metrics and monitoring
- Search engines for full-text search and complex queries
- Key-value stores for caching and simple data structures
Implementation challenges:
- Maintaining data consistency across database systems
- Creating a unified query interface
- Managing data duplication and synchronization
- Complexity in development and operations
- Monitoring across different database technologies
Real-World Optimization Examples
Let’s examine some practical optimization scenarios I’ve encountered in real projects.
Case Study 1: E-commerce Product Catalog
Challenge: A product catalog with 5 million items was experiencing slow search and filtering operations, with page load times exceeding 3 seconds.
Solution:
- Implemented strategic denormalization by creating a products_search table with pre-joined attributes
- Added composite indexes tailored to common search patterns
- Implemented a materialized view for common aggregations (products per category, brand, etc.)
- Added application-level caching with Redis for common queries
- Implemented cache invalidation triggered by product updates
Results:
- Search response time reduced from 3+ seconds to under 200ms
- Database load reduced by 70%
- Ability to handle 5x more concurrent users
Case Study 2: Financial Transaction System
Challenge: A financial application processing 500,000+ daily transactions was experiencing deadlocks and growing latency during peak hours.
Solution:
- Partitioned the transactions table by date
- Implemented read replicas for reporting queries
- Moved historical transactions (>90 days) to a separate archive database
- Optimized transaction isolation levels for different operation types
- Implemented connection pooling with proper sizing
Results:
- Eliminated deadlocks completely
- Reduced peak-time latency by 85%
- Improved backup and maintenance operations
- Enhanced ability to scale for future growth
Case Study 3: Content Management System
Challenge: A CMS with rich content types was struggling with complex content retrieval operations and slow content updates.
Solution:
- Implemented polyglot persistence:
- Kept structured metadata in PostgreSQL
- Moved textual content to Elasticsearch for advanced search
- Used Redis for caching and real-time features
- Created a unified API layer to abstract the underlying data stores
- Implemented an event-driven architecture to propagate updates across systems
Results:
- Content retrieval time reduced by 90%
- Complex searches became 40x faster
- System could now scale content without performance degradation
- Enhanced search capabilities with minimal development effort
Performance Monitoring and Optimization
Optimization is an ongoing process requiring continuous monitoring and refinement:
Essential Monitoring Metrics
Track these key database metrics:
- Query performance: Execution time, scan types, rows examined vs. returned
- Resource utilization: CPU, memory, disk I/O, network traffic
- Throughput: Queries per second, transactions per second
- Concurrency: Active connections, lock contention, deadlocks
- Cache efficiency: Hit rates, eviction rates, memory usage
Optimization Methodology
Follow a systematic approach to optimization:
- Establish baselines: Document normal performance patterns
- Identify bottlenecks: Use monitoring tools to pinpoint issues
- Prioritize improvements: Focus on high-impact, low-effort optimizations first
- Test changes: Verify improvements in staging before production
- Measure results: Quantify the impact of each optimization
- Iterate: Continue the cycle as application needs evolve
Tools of the Trade
Leverage these essential database optimization tools:
- Query analyzers: EXPLAIN, query plan visualizers
- Profilers: Database-specific profiling tools
- Monitoring systems: Time-series metrics collection and alerting
- Index analyzers: Tools to identify missing or unused indexes
- Load testing frameworks: Simulate production workloads
- APM (Application Performance Monitoring): End-to-end performance visibility
Conclusion: A Strategic Approach to Database Optimization
Database optimization should be viewed as a strategic initiative rather than a one-time fix. The most successful approach combines:
- Proactive design: Building performance considerations into data models and queries from the start
- Regular maintenance: Ongoing index optimization, statistics updates, and query refinement
- Scaling strategy: A clear roadmap for addressing increased data volumes and user loads
- Technology evolution: Willingness to adopt new database technologies when appropriate
- Performance culture: Making database performance a team-wide priority
By implementing the strategies outlined in this article—query optimization, effective indexing, intelligent caching, and appropriate scaling patterns—you can create web applications that remain responsive and reliable even as they grow to serve millions of users.
Remember that database optimization is both an art and a science. While these principles provide a foundation, each application has unique characteristics that may require custom approaches. The key is to understand your specific workload patterns, measure performance systematically, and apply targeted optimizations where they’ll have the greatest impact.
About the Author: Anthony Trivisano is a database optimization specialist with over 15 years of experience designing and optimizing data systems for web applications across various industries. He specializes in performance tuning, scalable architectures, and modernizing legacy database systems.