The State of Frontend Development in 2025: Frameworks, Tools, and Best Practices
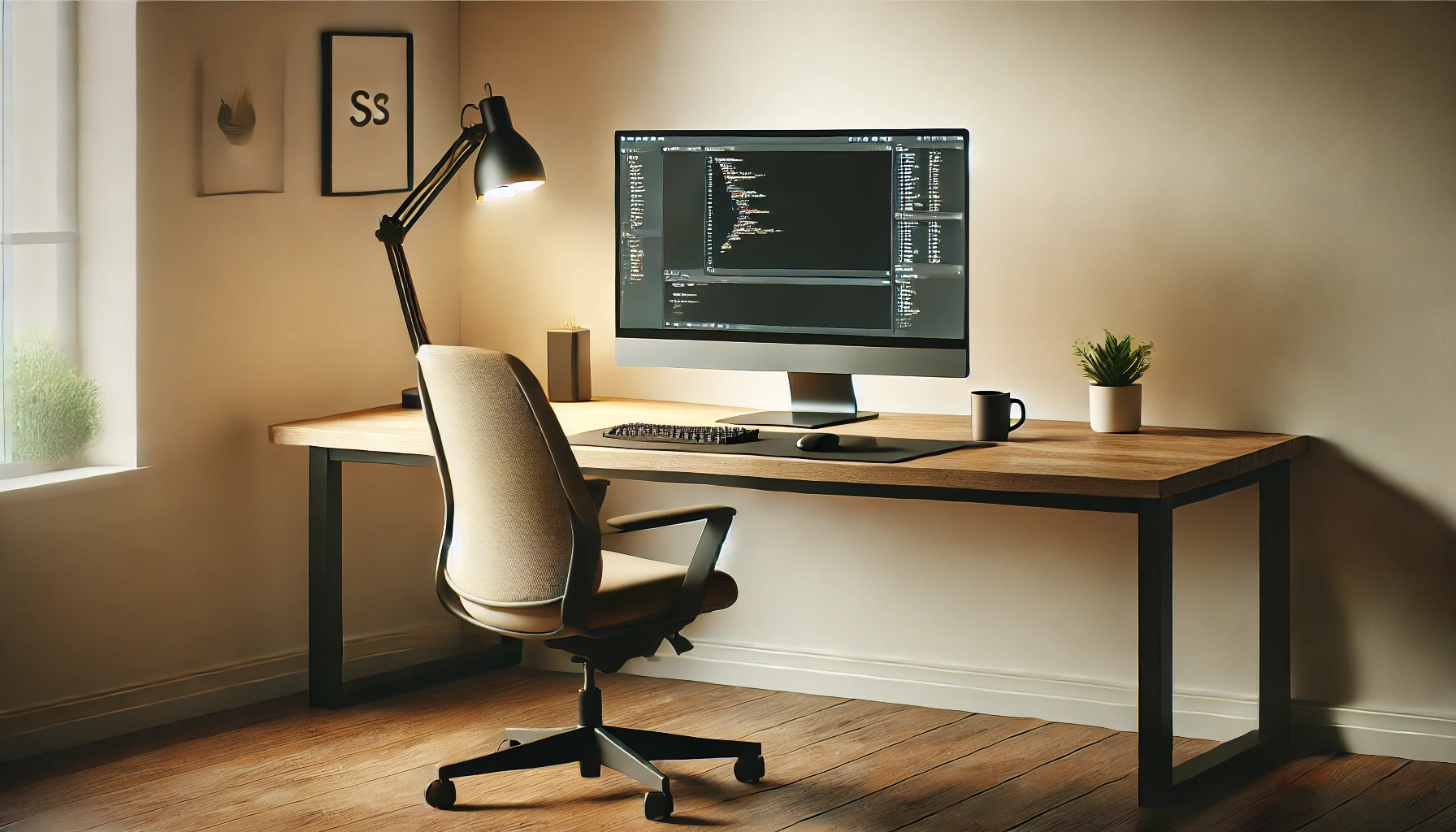
The State of Frontend Development in 2025: Frameworks, Tools, and Best Practices
Frontend development continues to evolve at a rapid pace. What began as simple HTML pages has transformed into complex applications with sophisticated architectures, powerful frameworks, and specialized tooling. As we navigate through 2025, the frontend landscape presents both exciting opportunities and significant challenges for developers and organizations.
In this comprehensive overview, I’ll examine the current state of frontend development, highlighting the most impactful frameworks, tools, and best practices based on my experience leading development teams across various industries.
The Evolution of JavaScript Frameworks
The JavaScript ecosystem has matured significantly, with frameworks becoming more specialized and catering to specific use cases rather than attempting to be all-encompassing solutions.
Component-Based Frameworks: The New Generation
React, Vue, and Angular have evolved substantially since their introduction, with each gaining distinctive strengths:
React has doubled down on its component model with significant improvements:
- The virtual DOM implementation has been further optimized for performance
- Server components have become mainstream, enabling more efficient rendering strategies
- The concurrent rendering model has matured, allowing for more responsive user interfaces
- React’s ecosystem continues to thrive with a wealth of libraries and tools
Vue has established itself as the balanced choice:
- Vue 4 brought significant performance improvements and better TypeScript integration
- The composition API has become the standard approach for complex applications
- Vue’s build tooling provides exceptional developer experience with minimal configuration
- The framework continues to excel at progressive enhancement and incremental adoption
Angular has successfully modernized while maintaining enterprise appeal:
- Angular’s signals-based reactivity system has replaced the traditional change detection
- Zone.js dependencies have been eliminated, improving performance and reducing complexity
- The framework’s developer experience has been streamlined while maintaining robust features
- Its comprehensive nature continues to appeal to enterprise applications
The Rise of Meta-Frameworks
Meta-frameworks built atop these foundation libraries have become the default starting point for many projects:
Next.js has expanded beyond React to become a comprehensive platform:
- The App Router pattern has fully matured with robust support for nested layouts
- Server components have become the default approach for most content
- The framework now includes built-in authentication, database integrations, and deployment options
- Vercel’s ecosystem continues to expand with complementary tools and services
Nuxt has evolved into a full-stack framework for Vue applications:
- Nuxt 4 introduced significant performance improvements and expanded server capabilities
- The framework now includes robust data fetching patterns and state management solutions
- Nitro server engine provides powerful server-side capabilities with minimal configuration
- The ecosystem has grown to include enterprise-grade features and integrations
Astro has pioneered a new approach to content-focused websites:
- Its “islands architecture” has inspired many frameworks to adopt partial hydration
- Expanded framework integrations allow developers to use multiple component libraries together
- Performance-first approach has set new standards for web performance
- Content collections and type safety have made it ideal for content-heavy applications
JavaScript Runtime Evolution
The JavaScript runtime environment itself has evolved significantly:
- Browser capabilities have expanded dramatically, with better support for modern JavaScript features
- Edge computing platforms allow JavaScript to run closer to users with near-native performance
- WebAssembly integration has become seamless, enabling high-performance calculations directly in the browser
- Worklet APIs provide optimized paths for animations, audio processing, and other performance-critical operations
Styling and Design Systems
The approach to styling web applications has transformed significantly from traditional CSS to more component-oriented solutions.
CSS Evolution
CSS itself has gained powerful new features that have reduced the need for preprocessors and frameworks:
- CSS Variables (Custom Properties) are now used extensively for theming and dynamic styling
- Container Queries have fundamentally changed responsive design, enabling truly modular components
- CSS Grid and Flexbox have become more sophisticated, with subgrid support now universal
- :has() selector has enabled previously impossible parent-based styling
- @layer and @scope directives provide better CSS organization and encapsulation
- Color functions like
oklch()
andcolor-mix()
enable more sophisticated color manipulation
Styling Methodologies
Several approaches to component styling have emerged as clear leaders:
CSS-in-JS has evolved significantly:
- Runtime performance issues have been addressed through compilation and extraction
- Zero-runtime CSS-in-JS libraries have become the standard
- Type safety and developer experience have improved dramatically
- Integration with design tokens and design systems has become seamless
Utility-first CSS continues to be popular:
- Tailwind CSS and similar frameworks have evolved to address criticisms about maintainability
- Just-in-time compilation is now standard, eliminating bundle size concerns
- IDE integrations provide excellent developer experiences with autocomplete and documentation
- Customization and extensibility have improved while maintaining performance
CSS Modules and Scoped CSS have remained relevant:
- Native CSS module support in browsers has eliminated many build-time complexities
- Scoped styles are now available natively in many frameworks
- Enhanced interoperability with design systems through variable-based theming
Design System Implementation
Design systems have become fundamental to frontend development:
┌─────────────────────────────────────────────────────┐
│ │
│ Design System │
│ │
├────────────┬─────────────┬───────────┬─────────────┤
│ │ │ │ │
│ Design │ Tokens │ Core │ Patterns │
│ Principles│ │ Components│ │
│ │ │ │ │
└────────────┴─────────────┴───────────┴─────────────┘
│ │
▼ ▼
┌────────────────────────┐ ┌─────────────────────────┐
│ │ │ │
│ Component Library │ │ Application-Specific │
│ │ │ Components │
│ │ │ │
└────────────────────────┘ └─────────────────────────┘
Key advancements include:
- Design tokens have become standardized, allowing seamless translation between design tools and code
- Automated accessibility checking is integrated directly into design systems
- Multi-brand support enables efficient management of multiple product lines
- Developer tooling provides guidance and validation when using design system components
- Version management allows controlled updates across large application portfolios
Performance Optimization
Web performance has become increasingly sophisticated, with new metrics, tools, and optimization techniques emerging.
Modern Performance Metrics
Performance measurement now focuses on user-centric metrics:
- Core Web Vitals have evolved beyond the original LCP, FID, and CLS
- Interaction to Next Paint (INP) has replaced First Input Delay as the key responsiveness metric
- Real User Monitoring (RUM) is now standard across production applications
- User experience scoring combines multiple metrics to evaluate overall performance
Advanced Optimization Techniques
Performance optimization has evolved beyond simple bundle size reduction:
Rendering Strategies have become more sophisticated:
- Partial Hydration enables interactive islands within mostly static content
- Progressive Hydration prioritizes critical interactions before loading non-essential features
- Resumability allows JavaScript execution to pause and resume without losing state
- Streaming Server Rendering delivers content to users as it becomes available
Resource Loading techniques have improved dramatically:
- Priority Hints allow developers to specify the importance of resources
- Script Loading Strategies have been refined based on extensive real-world data
- Speculative Loading preloads resources that might be needed based on user behavior
- Back/Forward Cache Optimization ensures instant navigation for previously visited pages
Image Optimization has become more automated:
- Next-generation formats like AVIF and WebP are used automatically based on browser support
- Responsive images are generated and served without developer intervention
- Content-aware cropping maintains visual focus across different aspect ratios
- Image CDNs have become integral to mainstream development workflows
State Management
As applications grow more complex, state management approaches have become more nuanced and specialized.
Reactive State Management
The landscape has evolved beyond the Redux vs. MobX debates:
- Signals-based reactivity has become mainstream across frameworks
- Atomic state management breaks state into smaller, composable pieces
- Server-centric state reduces the amount of client-side state management
- Framework-specific solutions like React’s Context API have matured
Server/Client State Separation
The distinction between server and client state has become clearer:
- Server state is handled through specialized data fetching libraries
- Form state has dedicated libraries optimized for validation and submission
- UI state is managed locally within components when possible
- Global application state is minimized and carefully segmented
┌─────────────────────────────────────────────────────┐
│ │
│ Application State │
│ │
├─────────────────┬─────────────────┬─────────────────┤
│ │ │ │
│ Server State │ UI State │ Client-only │
│ │ │ State │
│ │ │ │
├─────────────────┴─────────────────┴─────────────────┤
│ │
│ State Management Libraries │
│ │
└─────────────────────────────────────────────────────┘
Data Fetching Patterns
Data fetching has been refined and standardized:
- React Query/SWR patterns have become the standard approach for managing server state
- GraphQL adoption has stabilized, with specialized use cases benefiting from its capabilities
- Tightly coupled backend/frontend frameworks provide seamless data access patterns
- Edge computing has changed data fetch patterns by moving processing closer to users
Testing and Quality Assurance
Testing approaches have evolved to match the complexity of modern web applications:
Component Testing
Component testing has become more sophisticated:
- Component Test Runners provide tailored environments for testing UI components
- Visual Regression Testing is now standard for catching unexpected UI changes
- Interaction Testing verifies component behavior beyond simple rendering
- Accessibility Testing is integrated directly into component test suites
End-to-End Testing
End-to-end testing has become more reliable and efficient:
- Modern E2E frameworks like Playwright and Cypress have reduced flakiness and improved developer experience
- Component Testing within E2E frameworks bridges the gap between unit and integration tests
- AI-assisted test generation helps create comprehensive test coverage
- Performance testing is integrated into the E2E testing process
Continuous Integration
Testing is now deeply integrated into development workflows:
- Pull Request Previews automatically deploy changes for review
- Visual Comparison Tools highlight UI changes directly in pull requests
- Performance Budgets prevent performance regression
- Accessibility Reports ensure inclusive user experiences
Build Tooling and Development Experience
The development experience has improved dramatically through better tooling:
Build Systems
Build tools have become faster and more capable:
- Build-less development environments provide instant feedback during development
- Intelligent bundling optimizes output based on actual browser support requirements
- Partial rebuilds minimize the impact of changes during development
- Build caching dramatically speeds up repeated builds
Type Safety
Type systems have become integral to frontend development:
- TypeScript has become the standard language for larger applications
- JSDoc type annotations provide type safety for projects that prefer vanilla JavaScript
- API type generation automatically creates types from backend schemas
- Runtime type checking validates external data against type definitions
Developer Tools
Developer tooling has expanded beyond browser DevTools:
- IDE integrations provide rich feedback during development
- Automated refactoring tools assist with codebase maintenance
- Intelligent code suggestions powered by AI improve developer productivity
- Documentation generation creates and maintains documentation from code
Accessibility and Inclusive Design
Accessibility has shifted from an afterthought to a fundamental consideration:
Accessibility Integration
Accessibility is now built into the development process:
- Automated testing catches common accessibility issues during development
- Component libraries include accessibility features by default
- Design systems enforce accessible color contrast and interaction patterns
- Framework-specific tools ensure proper ARIA usage and keyboard navigation
Beyond WCAG Compliance
Accessibility now extends beyond technical compliance:
- Inclusive design practices consider a wide range of user needs and preferences
- Personalization options allow users to adapt interfaces to their specific requirements
- Reduced motion support protects users with vestibular disorders
- Cognitive accessibility considerations help users with cognitive disabilities
Emerging Trends and Future Directions
Several emerging trends are shaping the future of frontend development:
AI-Assisted Development
AI is transforming the development process:
- Code generation helps create boilerplate and common patterns
- Bug detection identifies potential issues before they reach production
- Performance optimization suggests improvements to existing code
- Accessibility improvements are suggested during development
Web Components and Standardization
Web standards continue to advance:
- Web Components have gained broader adoption for shareable UI elements
- Browser APIs continue to expand, reducing the need for JavaScript polyfills
- Progressive Web Apps have become more powerful with expanded capabilities
- WebGPU enables high-performance graphics processing directly in the browser
Sustainability and Ethical Development
Ethical considerations have become more prominent:
- Green web development practices reduce the environmental impact of web applications
- Privacy-first design prioritizes user data protection
- Inclusive teamwork ensures diverse perspectives in the development process
- Ethical AI usage considers the implications of AI-assisted development
Actionable Best Practices for 2025
Based on the current landscape, here are key recommendations for frontend development in 2025:
1. Adopt a Rendering Strategy, Not Just a Framework
Choose rendering approaches based on specific requirements:
- Static content: Use static generation where possible
- Dynamic content: Apply server rendering with selective hydration
- Interactive applications: Use client-side rendering with server components
- Hybrid approaches: Combine multiple rendering strategies within the same application
2. Optimize for Core Web Vitals
Make performance a primary consideration:
- Establish performance budgets for each vital metric
- Implement automated performance testing in your CI/CD pipeline
- Use RUM data to identify and address real-world performance issues
- Prioritize user-perceived performance over technical metrics
3. Embrace Type Safety
Type systems provide significant benefits:
- Use TypeScript for large applications and teams
- Consider JSDoc types for smaller projects
- Generate types from API schemas to ensure consistency
- Implement runtime validation for external data
4. Invest in a Design System
Design systems improve consistency and development efficiency:
- Start with design tokens and core components
- Implement accessibility requirements at the design system level
- Create clear documentation and usage guidelines
- Establish governance processes for design system evolution
5. Prioritize Accessibility from the Start
Accessibility should be a fundamental consideration:
- Include accessibility requirements in user stories
- Test for accessibility during development, not just before release
- Train developers in accessibility best practices
- Include people with disabilities in user testing
Conclusion: Navigating the Complexity
The frontend landscape in 2025 offers powerful capabilities but also presents significant complexity. Success requires thoughtful technology choices, a focus on fundamentals, and a commitment to continuous learning.
Rather than chasing every new framework or tool, focus on:
- Understanding principles over specific implementations
- Building robust architecture that can evolve over time
- Adopting tools that solve real problems rather than following trends
- Investing in developer experience to maintain productivity
- Prioritizing user needs over technical preferences
By focusing on these fundamentals while strategically adopting new technologies, you can create exceptional user experiences that perform well, remain maintainable, and adapt to changing requirements.
What frontend challenges is your organization facing? I’d be happy to discuss specific strategies for your particular context.
About the Author: Anthony Trivisano is a technology leadership consultant with extensive experience building and managing development teams. He specializes in web application architecture, performance optimization, and creating efficient development processes.