API Development Best Practices: Creating Robust and Scalable Web Services
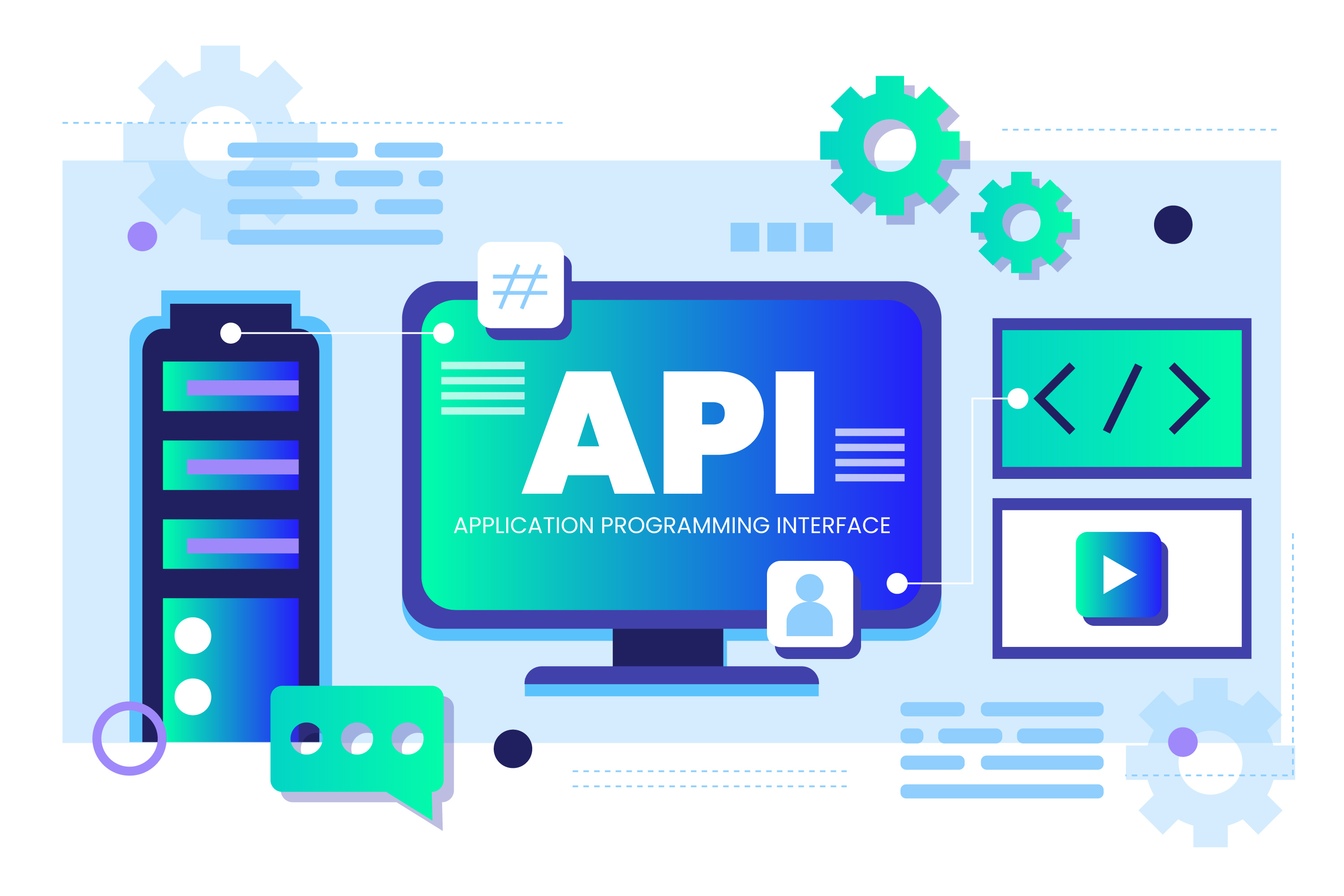
API Development Best Practices: Creating Robust and Scalable Web Services
Application Programming Interfaces (APIs) have become the foundation of modern web development, enabling seamless communication between different systems, services, and applications. A well-designed API isn’t just a technical implementation—it’s a product that serves both business needs and developer requirements.
Throughout my career leading development teams and implementing complex systems, I’ve found that successful APIs share certain characteristics: they’re intuitive to use, reliable under load, secure against threats, and flexible enough to evolve with changing requirements. In this article, I’ll share proven best practices for creating APIs that exhibit these qualities.
API Design Fundamentals
Before diving into specific implementation details, let’s explore the core principles that should guide your API design process.
API-First Development
The API-first approach prioritizes your API design before implementation begins:
- Design before implementation: Create a detailed API specification before writing code
- Contract-first mindset: Focus on the interface rather than implementation details
- Developer experience: Consider API consumers from the beginning
- Stakeholder involvement: Include product managers and potential consumers in the design process
This approach ensures that your API meets actual business and developer needs, rather than simply exposing your internal implementation.
Choosing the Right API Style
The two dominant API styles today are REST and GraphQL, each with distinct advantages:
REST (Representational State Transfer)
REST remains the most widely adopted API style, offering:
- Familiarity: Well-understood by most developers
- Simplicity: HTTP-based with clear resource modeling
- Cacheability: Effective use of HTTP caching mechanisms
- Tooling: Mature ecosystem of tools and libraries
A well-designed RESTful API follows these principles:
- Organize around resources (nouns, not verbs)
- Use standard HTTP methods appropriately (GET, POST, PUT, DELETE)
- Utilize HTTP status codes effectively
- Provide hypermedia links (HATEOAS) when appropriate
- Use consistent resource naming conventions
GraphQL
GraphQL offers an alternative approach that excels in certain scenarios:
- Query flexibility: Clients request exactly the data they need
- Reduced round trips: Multiple resources retrieved in a single request
- Strong typing: Schema provides clear contract and enables tooling
- Evolution: Add fields without creating new versions
- Real-time capabilities: Subscriptions for event-driven updates
GraphQL works especially well when:
- Clients need to retrieve deeply nested or related data
- Different client applications need varying data shapes
- Network performance is critical (mobile applications)
- Rapid iteration on frontend requirements is expected
Making the Choice
The decision between REST and GraphQL should consider your specific requirements:
┌───────────────────────────────────────────────────┐
│ │
│ Choose GraphQL when: │
│ │
│ • Clients need flexible, precise data fetching │
│ • Your data model has complex relationships │
│ • You have diverse client needs │
│ • Network efficiency is critical │
│ • Strong typing is highly valuable │
│ │
└───────────────────────────────────────────────────┘
┌───────────────────────────────────────────────────┐
│ │
│ Choose REST when: │
│ │
│ • Your use cases align with CRUD operations │
│ • HTTP caching is important │
│ • You need maximum tooling compatibility │
│ • Your team has REST expertise │
│ • Simpler client implementation is preferred │
│ │
└───────────────────────────────────────────────────┘
Many organizations use both approaches, selecting the right tool for each specific use case.
REST API Design Best Practices
If you’ve chosen REST for your API, follow these best practices to ensure a high-quality implementation.
Resource Modeling
The foundation of a good REST API is proper resource modeling:
- Identify key business entities: Map your domain model to API resources
- Use plural nouns for collection resources:
/users
rather than/user
- Apply consistent naming conventions: Use kebab-case or camelCase consistently
- Limit resource nesting: Avoid deeply nested URLs like
/users/123/orders/456/items/789
- Consider relationship resources: Use
/users/123/orders
to list a user’s orders
HTTP Methods
Use HTTP methods consistently for their intended purposes:
- GET: Retrieve resources (idempotent, safe)
- POST: Create new resources or trigger actions
- PUT: Update resources by replacing them entirely (idempotent)
- PATCH: Partially update resources (non-idempotent)
- DELETE: Remove resources (idempotent)
Examples of proper HTTP method usage:
GET /users # List users
GET /users/123 # Get user 123
POST /users # Create a new user
PUT /users/123 # Replace user 123 completely
PATCH /users/123 # Update some fields of user 123
DELETE /users/123 # Delete user 123
Query Parameters
Use query parameters effectively:
- Filtering:
/users?status=active
- Sorting:
/users?sort=last_name,-created_at
- Pagination:
/users?page=2&per_page=25
- Field selection:
/users?fields=id,name,email
- Search:
/users?q=smith
Establish consistent patterns for these parameters across all your API endpoints.
Status Codes
Use HTTP status codes to communicate outcomes clearly:
Success codes:
- 200 OK: Request succeeded
- 201 Created: Resource created successfully
- 204 No Content: Success with no response body
Client error codes:
- 400 Bad Request: Malformed request or invalid data
- 401 Unauthorized: Authentication required
- 403 Forbidden: Authentication succeeded but access denied
- 404 Not Found: Resource doesn’t exist
- 422 Unprocessable Entity: Validation errors
Server error codes:
- 500 Internal Server Error: Unexpected server error
- 503 Service Unavailable: Server temporarily unavailable
Use the most specific status code that applies to each situation.
Response Structure
Create consistent, well-structured responses:
{
"data": {
"id": "123",
"type": "users",
"attributes": {
"name": "Jane Smith",
"email": "jane.smith@example.com",
"created_at": "2025-01-15T14:30:21Z"
},
"relationships": {
"orders": {
"links": {
"related": "/api/users/123/orders"
}
}
}
},
"meta": {
"request_id": "a1b2c3d4"
}
}
Consider standards like JSON:API or HAL for a consistent approach to resource representation.
GraphQL API Design Best Practices
If GraphQL better fits your requirements, follow these practices for effective implementation.
Schema Design
The GraphQL schema is your API contract:
- Design schemas around domains: Align with your business concepts
- Use clear, descriptive types: Names should be self-explanatory
- Apply consistent naming conventions: CamelCase for fields, PascalCase for types
- Leverage scalar types: Use custom scalars for dates, emails, etc.
- Consider interfaces and unions: Model polymorphic relationships effectively
A well-structured GraphQL schema example:
type User {
id: ID!
name: String!
email: Email!
role: UserRole!
createdAt: DateTime!
orders(status: OrderStatus): [Order!]!
}
enum UserRole {
CUSTOMER
ADMIN
SUPPORT
}
type Order {
id: ID!
status: OrderStatus!
totalAmount: Money!
items: [OrderItem!]!
createdAt: DateTime!
}
scalar Email
scalar DateTime
scalar Money
Query Design
Optimize your GraphQL queries for usability and performance:
- Limit query depth: Prevent deeply nested queries that could impact performance
- Implement pagination: Use cursor-based pagination for collections
- Consider query complexity: Calculate and limit query complexity to prevent abuse
- Define sensible defaults: Make optional arguments truly optional with reasonable defaults
- Support partial responses: Allow clients to request only needed fields
Mutations
Structure mutations consistently:
- Use input types: Define input objects for mutation arguments
- Return modified resources: Include the affected resource in the response
- Handle errors granularly: Return field-level errors when possible
- Follow naming conventions:
createUser
,updateUser
,deleteUser
Example of a well-designed mutation:
type Mutation {
createUser(input: CreateUserInput!): CreateUserPayload!
}
input CreateUserInput {
name: String!
email: Email!
role: UserRole = CUSTOMER
}
type CreateUserPayload {
user: User
errors: [Error!]!
}
type Error {
path: [String!]!
message: String!
}
Performance Considerations
Address GraphQL-specific performance concerns:
- Implement batching: Use DataLoader pattern to avoid N+1 queries
- Consider caching: Implement field-level and response caching strategies
- Use persisted queries: Cache frequently used queries by ID
- Apply query cost analysis: Calculate and limit query cost
- Optimize resolver implementation: Make resolvers efficient and focused
Authentication and Authorization
Security is paramount for any API. Implement these practices to protect your API and its users.
Authentication Strategies
Choose the right authentication mechanism for your needs:
- API keys: Simple but limited security for internal or partner APIs
- OAuth 2.0: Industry standard for delegated authorization
- JWT (JSON Web Tokens): Stateless authentication with signed tokens
- OpenID Connect: Identity layer on top of OAuth 2.0
- Session-based authentication: Traditional approach using server-side sessions
Each approach has different security implications and implementation complexity.
JWT Best Practices
If using JWTs for authentication:
- Keep tokens secure: Store securely on clients, use HTTPS exclusively
- Include minimal data: Don’t bloat tokens with unnecessary information
- Set appropriate expiry: Balance security and user experience
- Implement refresh token flows: Allow session extension without frequent re-authentication
- Use signing algorithms properly: Prefer RS256 over HS256 for production
- Consider token revocation: Implement a revocation strategy if required
Authorization Implementation
Authentication (who you are) is just the beginning; authorization (what you can do) is equally important:
- Role-based access control (RBAC): Assign permissions to roles, then roles to users
- Attribute-based access control (ABAC): Base permissions on attributes of users, resources, and context
- Resource-level permissions: Control access to specific resources or collections
- Action-level permissions: Differentiate permissions for reading vs. modifying data
- Field-level security: Control access to specific fields within resources
Authorization should be enforced at the API gateway or service layer, never relying solely on client-side checks.
Example of implementing authorization with middleware:
// Express.js example
const checkPermission = (requiredPermission) => {
return (req, res, next) => {
const user = req.user; // From authentication middleware
if (!user || !hasPermission(user, requiredPermission)) {
return res.status(403).json({
error: 'Forbidden',
message: 'You do not have permission to perform this action'
});
}
next();
};
};
// Route with authorization
app.patch('/users/:id',
authenticate,
checkPermission('users:update'),
userController.update
);
Security Best Practices
Beyond authentication and authorization, implement these security measures:
- Always use HTTPS/TLS: Encrypt all API traffic
- Implement rate limiting: Prevent abuse and denial of service attacks
- Validate all input: Guard against injection attacks and invalid data
- Set appropriate CORS headers: Control cross-origin access
- Use security headers: Implement HTTP security headers (Content-Security-Policy, etc.)
- Prevent parameter pollution: Handle duplicate query parameters properly
- Monitor for suspicious activity: Log and alert on unusual patterns
- Keep dependencies updated: Regularly update libraries to address vulnerabilities
API Documentation
Great documentation is essential for API adoption and proper usage.
Documentation Types
Provide multiple forms of documentation:
- Reference documentation: Complete technical details of all endpoints
- Guides and tutorials: Task-oriented documentation for common scenarios
- Examples and use cases: Real-world examples showing correct usage
- API playground: Interactive environment to experiment with the API
- SDKs and client libraries: Code libraries that simplify API consumption
- Changelog: History of API changes and version compatibility
OpenAPI/Swagger
For REST APIs, OpenAPI (formerly Swagger) has become the standard for documentation:
- Machine-readable specification: Create an OpenAPI document describing your API
- Human-readable docs: Generate interactive documentation from the specification
- Code generation: Create client libraries and server stubs from the specification
- Validation: Validate requests against the schema
- Mock servers: Generate test servers from your specification
# OpenAPI example (partial)
openapi: 3.0.0
info:
title: User Management API
version: 1.0.0
description: API for managing users and their orders
paths:
/users:
get:
summary: List all users
parameters:
- name: status
in: query
schema:
type: string
enum: [active, inactive, pending]
responses:
'200':
description: List of users
content:
application/json:
schema:
$ref: '#/components/schemas/UserList'
GraphQL Schema Documentation
For GraphQL APIs, leverage the self-documenting nature of the schema:
- Schema introspection: Enable introspection in non-production environments
- GraphiQL or GraphQL Playground: Provide interactive schema explorers
- Schema documentation strings: Add detailed descriptions to types and fields
- Examples in documentation: Show sample queries and responses
- Usage guidelines: Document best practices specific to your API
Versioning and Evolution
APIs need to evolve while maintaining backward compatibility for existing clients.
Versioning Strategies
Several approaches to API versioning exist:
- URL path versioning:
/api/v1/users
,/api/v2/users
- Query parameter versioning:
/api/users?version=2
- Header-based versioning:
Accept: application/vnd.company.v2+json
- Content negotiation:
Accept: application/json; version=2
- Unversioned with backward compatibility: Evolve without breaking changes
Each approach has trade-offs in terms of visibility, client implementation complexity, and caching implications.
Evolving APIs Without Breaking Changes
When possible, evolve your API without requiring clients to update:
- Add new fields and endpoints: Addition is generally non-breaking
- Make required fields optional: Relax constraints rather than tightening them
- Use enum extensibility pattern: Allow for new enum values
- Implement feature toggles: Enable gradual rollout of changes
- Provide default behavior: Ensure sensible defaults for new parameters
- Maintain old fields alongside new ones: Use deprecation for transition periods
Managing Breaking Changes
When breaking changes are necessary:
- Announce well in advance: Give clients time to adapt
- Provide clear migration guides: Document exactly what needs to change
- Offer side-by-side availability: Run old and new versions simultaneously during transition
- Set and communicate deprecation timelines: Establish clear end-of-life dates
- Monitor version usage: Track which clients use which version
Performance and Scaling
A well-designed API must perform well under load and scale as demand increases.
Performance Optimization
Implement these techniques to optimize API performance:
- Efficient database queries: Optimize queries and use appropriate indexes
- Connection pooling: Reuse database connections
- Caching strategies: Implement HTTP caching, application caching, and database query caching
- Compression: Use gzip/brotli compression for responses
- Asynchronous processing: Move long-running tasks to background jobs
- Pagination and limits: Control resource consumption with sensible defaults
- Optimized serialization: Use efficient data formats and serialization methods
Scaling Strategies
Prepare your API for growth with these scaling approaches:
- Horizontal scaling: Add more instances behind a load balancer
- Vertical scaling: Increase resources on existing instances
- Microservices architecture: Split large APIs into focused services
- Serverless deployment: Use cloud functions for variable workloads
- Edge caching: Deploy to CDNs and edge locations for global performance
- Database sharding: Partition database for increased capacity
- Read replicas: Offload read operations to database replicas
Rate Limiting and Throttling
Protect your API resources with proper limits:
- Request rate limiting: Limit requests per client per time period
- Concurrency limiting: Control simultaneous requests
- Quota management: Set usage limits by time period or features
- Resource-based throttling: Apply different limits to different endpoints
- Graceful degradation: Return appropriate status codes (429 Too Many Requests)
- Backoff guidance: Provide retry-after headers
Example rate limiting implementation:
// Rate limiting middleware with Redis
const rateLimit = require('express-rate-limit');
const RedisStore = require('rate-limit-redis');
const apiLimiter = rateLimit({
store: new RedisStore({
client: redisClient
}),
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // Limit each IP to 100 requests per windowMs
standardHeaders: true, // Return rate limit info in the headers
legacyHeaders: false, // Disable the X-RateLimit-* headers
handler: (req, res) => {
res.status(429).json({
error: 'Too Many Requests',
message: 'You have exceeded the rate limit',
retryAfter: Math.ceil(req.rateLimit.resetTime / 1000)
});
}
});
// Apply to all requests
app.use('/api/', apiLimiter);
Monitoring and Observability
Understanding how your API performs in production is critical for maintaining quality and addressing issues.
Metrics to Track
Collect and analyze these key metrics:
- Request volume: Requests per second, by endpoint
- Error rates: Percentage of requests resulting in errors, by type and endpoint
- Response times: Latency percentiles (p50, p95, p99)
- System resource usage: CPU, memory, disk, network utilization
- Dependency performance: Database query times, external API call latencies
- Business metrics: Transactions, conversions, or other domain-specific metrics
Logging Best Practices
Implement effective logging for troubleshooting:
- Structured logging: Use JSON or another structured format
- Correlation IDs: Include trace IDs that follow requests through the system
- Context details: Log relevant request context (user, endpoint, parameters)
- Error details: Include stack traces and error contexts
- Sensitive data handling: Mask or exclude sensitive information
- Log levels: Use appropriate severity levels (INFO, WARN, ERROR)
Distributed Tracing
For microservice architectures, implement distributed tracing:
- Trace context propagation: Pass trace IDs between services
- Span collection: Record timing for each service and operation
- Service dependency mapping: Visualize the relationships between services
- Performance analysis: Identify bottlenecks across the distributed system
- Error correlation: Connect errors across multiple services
Testing Strategies
Comprehensive testing ensures API quality and reliability.
Testing Levels
Implement testing at multiple levels:
- Unit testing: Test individual functions and methods
- Integration testing: Test interactions between components
- Contract testing: Verify API conforms to its specification
- Functional testing: Test complete API behaviors
- Load testing: Verify performance under expected and peak loads
- Security testing: Identify vulnerabilities and security issues
Automation and CI/CD
Integrate API testing into your development pipeline:
- Automated test suites: Run tests automatically with each build
- Test environments: Maintain dedicated environments for testing
- Service virtualization: Mock external dependencies for testing
- Continuous integration: Test code changes immediately
- Continuous deployment: Automatically deploy verified changes
- Blue/green deployments: Deploy without downtime and verify before switching traffic
API Testing Tools
Leverage these types of tools for comprehensive API testing:
- HTTP clients: Tools like Postman, Insomnia, or curl for manual testing
- Testing frameworks: Jest, Mocha, pytest, etc. for automated testing
- Contract testing tools: Tools like Pact for consumer-driven contract testing
- Load testing tools: k6, JMeter, or Artillery for performance testing
- Security scanning tools: OWASP ZAP, Burp Suite for security testing
- API monitors: Continuous verification in production environments
Error Handling
Effective error handling helps both API developers and consumers quickly identify and resolve issues.
Error Response Structure
Provide clear, actionable error responses:
{
"error": {
"code": "VALIDATION_ERROR",
"message": "The request data is invalid",
"details": [
{
"field": "email",
"message": "Must be a valid email address"
},
{
"field": "password",
"message": "Must be at least 8 characters long"
}
],
"traceId": "abc-123-xyz",
"documentation": "https://api.example.com/docs/errors#VALIDATION_ERROR"
}
}
Include these elements in error responses:
- Error code: Unique identifier for the error type
- User-friendly message: Clear explanation of what went wrong
- Detailed information: Specific issues, preferably field-by-field
- Request identifier: Correlation ID for tracing and support
- Documentation link: Further information about the error and resolution
Error Types
Handle different categories of errors appropriately:
- Validation errors: Issues with the request data (400 Bad Request)
- Authentication errors: Missing or invalid credentials (401 Unauthorized)
- Authorization errors: Insufficient permissions (403 Forbidden)
- Resource errors: Requested resource not found or unavailable (404 Not Found, 410 Gone)
- Conflict errors: Request conflicts with current state (409 Conflict)
- Rate limiting errors: Too many requests (429 Too Many Requests)
- Server errors: Internal issues (500 Internal Server Error)
- Service unavailable errors: Temporary unavailability (503 Service Unavailable)
Error Handling Best Practices
Follow these principles for robust error handling:
- Be specific: Provide detailed information about what went wrong
- Don’t leak internals: Avoid exposing implementation details or sensitive data
- Be consistent: Use the same error structure across all endpoints
- Fail early: Validate input before processing
- Log appropriately: Ensure errors are properly logged for debugging
- Consider partial success: For batch operations, report both successes and failures
API Gateways and Management
For production APIs, consider using API gateways and management platforms.
API Gateway Capabilities
API gateways provide critical infrastructure for API deployments:
- Request routing: Direct traffic to appropriate backend services
- Authentication and authorization: Centralized security enforcement
- Rate limiting and throttling: Protect backend resources
- Request/response transformation: Modify payload formats as needed
- Caching: Improve performance for frequently requested data
- Analytics and monitoring: Track API usage and performance
- Developer portal integration: Self-service documentation and key management
API Management Features
Consider these capabilities when selecting an API management solution:
- API lifecycle management: Control versions and deprecation
- Developer onboarding: Self-service registration and key management
- Usage plans and billing: Monetization and quota management
- Analytics and reporting: Insight into API usage patterns
- Documentation hosting: Centralized API documentation
- Community features: Forums, support, and feedback channels
- Governance and compliance: Policy enforcement and audit trails
Building an API Program
For organizations building multiple APIs, establish a cohesive API program:
API Governance
Implement governance to ensure consistency and quality:
- API design guidelines: Establish standards for all APIs
- Review processes: Ensure APIs meet quality standards before release
- Shared components: Create reusable patterns and implementations
- API catalog: Maintain an inventory of all available APIs
- Compliance verification: Ensure adherence to standards and regulations
Developer Experience
Prioritize the experience of API consumers:
- Intuitive design: Create APIs that are easy to understand and use
- Comprehensive documentation: Provide clear, complete documentation
- Predictability: Follow consistent patterns across all APIs
- Self-service capabilities: Enable developers to onboard without assistance
- Support channels: Offer ways for developers to get help when needed
- Feedback mechanisms: Collect and act on developer input
API as a Product
Treat your APIs as products with their own lifecycle:
- Clear value proposition: Understand what problem your API solves
- Target audience: Know who will use your API and why
- Roadmap: Plan future development based on user needs
- Metrics and KPIs: Measure success through meaningful metrics
- Feedback loop: Continuously improve based on user feedback
- Deprecation strategy: Plan for eventual replacement or retirement
Case Studies: API Design in Practice
Let’s examine how these principles apply in real-world scenarios.
Case Study 1: E-commerce API
Challenge: Build an API to support a multi-channel e-commerce platform with web, mobile, and partner integrations.
Solution:
- Implemented RESTful API for product catalog, orders, and customer management
- Used GraphQL for product search and personalized recommendations
- Applied OAuth 2.0 with different scopes for customers and partners
- Implemented rate limiting based on client tier
- Created comprehensive documentation with interactive examples
Results:
- Reduced mobile app load times by 40% through optimized data fetching
- Enabled partners to onboard in days rather than weeks
- Improved development velocity with clear contracts between teams
- Maintained 99.99% uptime while scaling to handle holiday traffic surges
Case Study 2: Financial Services API
Challenge: Create a secure, compliant API for a financial services company handling sensitive customer data.
Solution:
- Implemented strict authentication using OAuth 2.0 with PKCE
- Added fine-grained authorization with attribute-based access control
- Implemented comprehensive audit logging
- Applied multiple layers of input validation
- Created separate development, testing, and production developer portals
Results:
- Successfully passed security and compliance audits
- Reduced integration time for partners by 60%
- Maintained security with zero incidents despite high-value target status
- Scaled to handle 10x initial transaction volume without performance degradation
Conclusion: Building APIs That Last
Creating a successful API requires balancing many factors: technical excellence, business alignment, developer experience, and operational robustness. The practices outlined in this article represent hard-won lessons from years of API development across various industries.
Remember these key principles as you build your own APIs:
- Design intentionally: Consider your API’s design carefully before implementation
- Prioritize developer experience: Make your API intuitive and well-documented
- Build for evolution: Plan for change from the beginning
- Focus on security: Implement multiple layers of protection
- Measure everything: Understand how your API is used and performs
- Treat APIs as products: Align with user needs and business goals
By following these practices, you can create APIs that stand the test of time—delivering value to both your organization and your API consumers for years to come.
As the API landscape continues to evolve, the fundamental principles of good design remain constant. Focus on creating APIs that solve real problems, delight developers, and provide a solid foundation for your digital ecosystem.
About the Author: Anthony Trivisano is a technology leader with extensive experience designing and implementing APIs for organizations across various industries. He specializes in creating scalable, maintainable systems that align technical solutions with business objectives.